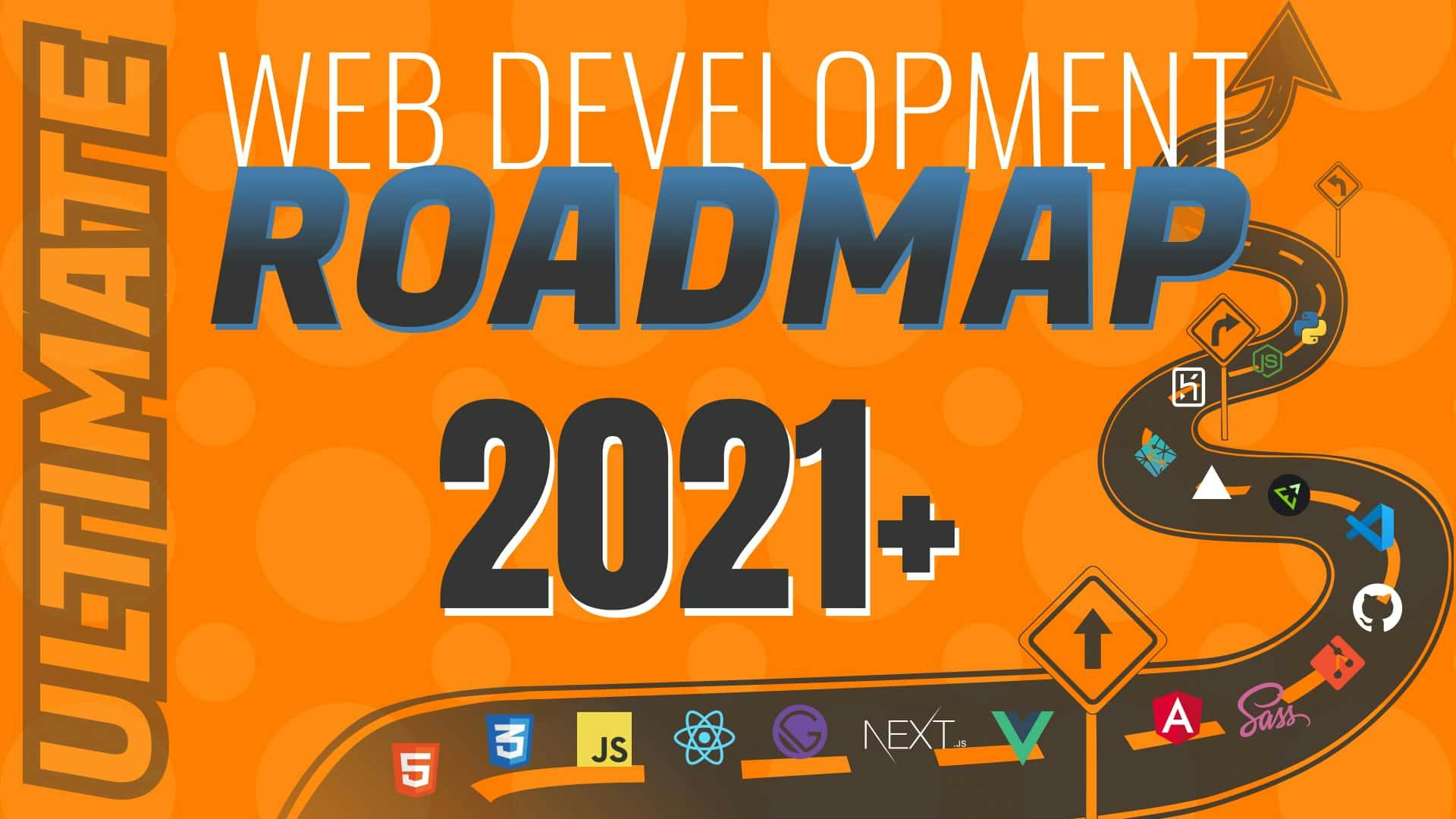
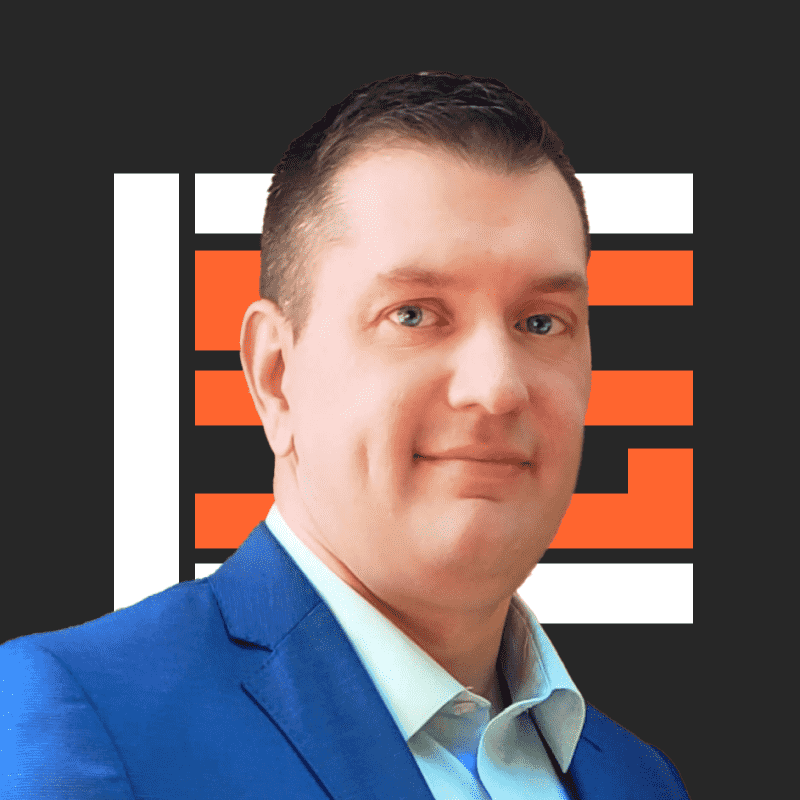
24 min read
Ultimate Guide to Web Development in 2021 & Beyond! | Roadmap 2021+
In this article, I'll guide you through my ultimate web development roadmap for 2021 and beyond.
We are going to cover a ton of technologies in this article. Don't get overwhelmed. You do not have to learn everything in this article.
This is an entire map of web development. Most web developers specialize in small parts of this. Such as UI/UX Design, Front-End development, Back-End development, CI/CD and DevOps.
What I hope is that this article will give you high-level picture of everything that is out there and then you can decide which route you want to take.
Not only am I going to tell you all of the technologies but also how and where to learn them.
There is a link in the description below to a FREE PDF that you can download. It will have links to all of the resources that I'll mention in this article. So be sure to download the PDF for reference.
๐ก Quick disclaimer: If I leave anything out of this roadmap, let me know in the description. I tried to think of everything, but I'm sure I missed something.
How The Internet Works
First, you need to understand how the internet works. You need a basic understanding of how computers communicate by using browsers, domain names, hosting services, HTTP/HTTPS, and DNS.
Resources:
- How the Internet Works in 5 Minutes (Aaron) - This is an old video, but it's still relevant.
- What is the Internet? (Code.org)
Basic Tools
๐ก Note: Anything with a ๐ denotes my personal favorite.
Browser
Browsers are a very important tool for web developers. They are the means of which end users will view our applications. I prefer to use Chrome as my main browser for testing, but you will need to install all of the major browsers to ensure that your application will work properly in all of them.
Text Editor
The text editor is a web developers best friend. You need to understand everything that it can do to help you write code as effeciently, accurately, and fast as possible.
My favorite code editor is VS Code! It is the most popular for the vast majority of developers.
I have a VS Code playlist and even an entire course that will help you Become A VS Code SuperHero!
Terminal
You will need to get used to using the terminal. Some are scared of it, but I promise, it's not that bad.
There are many options for a terminal. Every OS comes with a terminal. Windows has the Command Prompt and Powershell. You can also use the Windows Subsystem for Linux (WSL) which gives you a Linux shell but it's not the easiest thing to get setup and there are some limitations.
If you are on Windows, I would recommend using Git Bash. That's what I use. If you are on macOS or Linux/Unix, I would recommend Zsh.
Design
If you want to be a UI/UX designer, you will need to learn a design tool. I prefer Figma, but Adobe XD is great as well. Other options are Sketch, Illustrator, and Photoshop.
For anything design related, these are the 2 YouTube channels that I go to:
HTML
Every webpage uses HTML. It is the main building block of the web. HTML provides the structure of a website. It is actaully not a programming language, but a markup language.
You'll need to learn the basic syntax, semantic HTML, forms & validation, accessibility, SEO, and best practices.
Resources:
CSS
CSS is another important part of every website. It provides its styling, makes things look good on all devices, and adds flair with transitions and animations.
I'm not going to sugar coat this. There is a lot to CSS. If you are going to be a Front-End developer, it is very important that you understand CSS fully!
After learning the basics, you'll want to learn the layout features Flexbox and CSS Grid. Custom Properties are equivelent to variables in other languages. And, of course, transitions and animations are just really cool.
Animations can be done with plain CSS. But after you learn JavaScript, you can look into JavaScript libraries like GSAP (GreenSock Animation Platform) and anime.js. These make building complex animations super simple.
After that, be sure to get comfortable with responsive design including the mobile first approach, rem units, viewport settings, fluid widths, and media queries. You can learn all about this from the King of CSS, Kevin Powell.
The next three items you can come back to at any time. These are not necessary to learn but are great to understand.
Once you've master all of that you can move on to CSS pre-processors like Sass and PostCSS. I really love Sass. It gives your CSS superpowers!
If you start learning React, you'll most likely want to learn either styled components or CSS Modules.
Then there's CSS Frameworks. Some people just skip over CSS and go straight to frameworks. I HIGHLY discourage that. You need to have a solid understanding of CSS before learning a framework.
The 2 frameworks that I think are worth learning are Tailwind CSS and Bootstrap. They both have their places in web development.
Resources:
- CSS Crash Course in 30 Minutes (codeSTACKr)
- Learn CSS Flexbox in 20 Minutes (codeSTACKr)
- Learn CSS Grid in 20 Minutes (codeSTACKr)
- Learn Sass in 30 Minutes (codeSTACKr)
- GSAP Typing Animation (codeSTACKr)
- CSS King, Kevin Powell
Time to Choose
At this point, it's time to choose your path. Do you want to become a Front-End, Back-End, mobile, or desktop developer, or work with machine learning & AI?
It's important for me to note that even though I do have this broken up into sections, the lines between Front-End, Back-End, DevOps, etc, are getting blured.
My advice is to pick out the technologies that most interest you, no matter which path they are on. There are many companies that look for individuals that specialize in very small portions of each path.
So pick something and get good at it. It's better to be really good at one thing than to be meh at everything! ๐
Really quick, hit that like button to help me out and subscribe if you haven't already!
Front-End Path
Front-End development involves building the interface that the user sees in their browser. Generally, this includes HTML, CSS, and JavaScript. You'll work with Designers and Back-End developers mostly.
Designers will tell you what it should look like and how it should flow. Back-End developers will provide access to data, such as products, that will be shown on the website.
JavaScript
JavaScript is the first programming language that you'll learn as a Front-End developer. It makes the website do "things".
You'll need to learn the basic syntax, DOM manipulation, the fetch API, JSON, and ES6+ features.
You should spend most of your time on this part. It is extremely important that you fully understand JavaScript before moving on.
For those who want to learn a JavaScript framework like React or Vue, DO NOT start learning a framework before you understand "vanilla" JavaScript. These frameworks are based on JavaScript. You need this knowledge first.
Resources:
- JavaScript Tutorial for Beginners (codeSTACKr)
- Learn JSON in 10 Minutes (codeSTACKr)
- Fetch API Explained (codeSTACKr)
- JavaScript Playlist (codeSTACKr)
- JavaScript Shorts (codeSTACKr)
TypeScript
TypeScript is a superset of JavaScript. It allows for static typing. This helps you to catch errors and fix them before running your code.
When you use TypeScript, it will be compiled into regular JavaScript on build. So, this is a tool that you can learn at any point to make your code even better.
TypeScript can also be used with most frameworks.
Resources:
Front-End Frameworks
๐ก Note: I'm not going to get into the framework vs library discussion here. Whether something is technically a framework or a library is a moot point.
Front-End frameworks have many advantages. You can build powerful single page applications (SPA), keep your components organized, and easly build interactive UIs.
When you are working with a team, it becomes apparent how easy it is to break up an application into components for each team member to work on.
There are many frameworks available, but I'm only going to cover the top 4. (My opinion)
- React
- React is by far my personal favorite. It is fairly easy to learn and has a great community.
- Vue
- Vue is probably the easiest framework to learn.
- Angular
- Angular is a very popular framework, but I personally do not enjoy working with it. I beleive that it is more suited to very large applications.
- Svelte
- Svelte I believe is underrated. I would not use it for every application, but it does have it's place. And I plan on learning more about it in 2021.
Resources:
- React Playlist (codeSTACKr)
- Vue (Program With Erik)
- Vue (Faraday Academy)
- Angular Crash Course (Traversy Media)
- Svelte Crash Course (Traversy Media)
State Management
When you are working with frameworks, you'll soon realize how impotant it is to keep track of your applications state.
When you are dealing with many components and trying to pass data and state between them, you'll want to look into state management.
- Context API and Hooks (React)
- Redux (React)
- Vuex (Vue)
- Services (Angular)
Resources:
- React State Management Tutorial (Dev Ed)
- Redux For Beginners (Dev Ed)
- LEARN VUEX IN 15 MINUTES (Program With Erik)
Static Site Generators and Server Side Rendering (SSR)
Static site generators became very popular in 2020 and will continue in 2021. These make building simple things like landing pages so easy. But they are not just limited to that. A common use for SSG's are blogging sites.
With static site generators, you get better SEO, file system routing, and great plugin support from the community. Some use GraphQL for data fetching, such as Gatsby.
The most popular static site generators in my opinion are:
- Gatsby (React)
- Next.js (React)
- Gridsome (Vue)
- Nuxt.js (Vue)
- 11ty (I've been hearing a lot about this one but have to had time to dig into it.)
Server side rending (SSR) provides many of the same benifits of static sites but moves all rendering onto the server.
The current kings in this space are Next.js and Nuxt.js in my opinion.
You'll notice that Next.js and Nuxt.js can do both, static site generation and SSR. These are great options if you need flexibility. I'll be diving deeper into Next.js in 2021.
Resources:
- Gatsby (Weibenfalk)
- Next.js (Colby Fayock)
- Gridsome (Traversy Media - Gwen Faraday)
- Nuxt.js (Traversy Media)
More Tools
There are many more tools that you will need to learn. Here are the main ones.
- Package managers allow you to easily add pre-built packages into your application. These packages help you to complete certain functions within your application. I use npm. Others use yarn.
- Build Tools
- Task Runners Task runners help you in development by getting your environment up and running. They are also used during the build phase to perform tasks such as tree-shaking, minifying code, optomizing images, etc. - npm scripts - Gulp (Coder Coder)
- Linters & Formatters Linters and formatters help you to identify coding errors and formatting issues in your code. - Prettier - ESLint
- Module Bundlers Front-End frameworks use module bundlers to compile the code you have written down to regular HTML, CSS, and JavaScript. Many of these are built-in and require very little configuration on your part. But it is good to understand how these work. - Webpack (freeCodeCamp) - Parcel (James Q Quick) - Rollup
- Version control is very important. You will have to learn Git and GitHub, especially if you are working with a team. These allow you to keep track of changes, collaborate on projects, and are an integral part of CI/CD (Continuous Integration/Continuous Deployment) For everything open source and GitHub related, I look to Eddie Jaoude!
- You will be using your browsers' dev tools frequently. Be sure to get familiar with their capabilities. There are also specific browser extensions that can help with troubleshooting front-end frameworks like React.
- VS Code extensions are a must. There are many extensions that can help you with various technologies. Here is a video of my top extensions.
- If you are writing a lot of HTML and CSS, you have to learn Emmet! It will speed up your coding time dramatically! It's like coding shorthand.
- If you are dealing with a lot of data fetches, you may want to check out Axios. It's a nice JavaScript library that is easy to use instead of the standard Fetch API in JavaScript.
Other Technologies
GraphQL
GraphQL is a query language for APIs. It makes consuming data from your API on the client side so much nicer! It is built into Gatsby.
Progressive Web Apps (PWA)
PWAs are reliable and fast applications. They are most known for thier ability to be "installed" on devices like a regular application. But they are just web apps that give the user a native app experience without taking up space on thier device. One of their main features is the ability to work offline by using service workers.
WebAssembly (Wasm)
WebAssembly allows you to use other programming languages in the browser. There are other languages that are much faster than JavaScript. But keep in mind that this is not a replacement for JavaScript though.
So, if you are trying to build a next-level game or really complex tool that needs to cruch a lot of data, you'll want to look at WebAssembly.
Several languages that you can use with WebAssembly are C, C++, C#, and Rust.
JAMstack
The JAM in JAMstack stands for JavaScript, API, & Markup.
An example of a JAMstack would be the combination of Gatsby and Sanity.io. Sites that adhere to JAMstack guidelines are highly performant, cheap, and easy to scale. They are also super easy to host using Netlify or Vercel.
For more information on JAMstack visit jamstack.org.
Resources:
Speech Recognition
Speech recognition is only going to get more popular as time goes on. So many people are now used to talking to Alexa, Google, or Siri.
The fact is that people prefer to spend less time typing things. That is why voice searching and navigation will become even more popular in 2021.
You can implement speech recognition in your application using several technologies:
Resources:
Back-End Path
Working as a back-end developer involves managing servers, deployment, APIs, and databases mostly.
Pick a Language
- JavaScript (Node.js & Deno)
- Python
- Rust
- C#
- Java
- PHP
- Go
- Ruby
Picking a language depends on the purpose of the application being built. If you are working with large data sets, you may want to go with Python. If you just need a simple web server and you already know JavaScript, go for Node.js.
If you already have a company in mind that you want to work for, find out what they use on the back-end and learn that.
In the end, once you have learned one programming language it will be much easier to learn another. So don't sweat it too much.
Resources:
- Node.js Crash Course (Traversy Media)
- Deno Intro & Tutorial (codeSTACKr)
- Caleb Curry
- Rust Crash Course (Traversy Media)
- C# Full Course for Beginners (freeCodeCamp)
- Intro to Java (freeCodeCamp)
- PHP Tutorial (freeCodeCamp)
- Go Tutorial (freeCodeCamp)
- Ruby Full Course (freeCodeCamp)
Back-End Frameworks
The back-end has frameworks too. The same rules apply when learning a back-end framework. Learn the base language before jumping into the framework.
- Larvel (PHP)
- Spring (Java)
- Ruby on Rails (Ruby)
- Express (Node.js)
- Koa (Node.js)
- Django (Python)
- Flask (Python)
- Blazor (C#)
Resources:
- Laravel Crash Course 2020 (Traversy Media)
- Spring Boot Tutorial (freeCodeCamp)
- Ruby on Rails Crash Course 2020 (Traversy Media)
- Express JS Crash Course (Traversy Media)
- Django (Dennis Ivy)
- Learn Flask for Python (freeCodeCamp)
- Blazor (Claudio Bernasconi)
Databases
Just about every site has some data that it needs to keep. Examples of data would be products for sale or blog posts.
There are 2 main categories that databases fall into; Relational and NoSQL / Cloud.
Relational Databases
Relational databases are a lot like spreadsheets with tables. These table can link to each other using unique identifiers. Relational databases are more strict and require you to define each tables column and type ahead of time.
Examples of relational databases:
- PostgreSQL
- MySQL
- Azure Cloud SQL
- MS SQL
Resources:
NoSQL / Cloud Databases
NoSQL and cloud databases are not as strict as relational databases. You can define their data and types on the fly. It's important to understand these differences and pick the correct type of database that fits the needs of your application.
While NoSQL and cloud databases may be easier to get up and running at first, you may run into issues down the road as your database grows.
Examples of NoSQL & cloud databases:
Resources:
- MongoDB Crash Course (Traversy Media)
- FaunaDB Basics (Fireship)
- Firebase Beginner's Guide (Fireship)
- Build a React App with Airtable (codeSTACKr)
APIs
Now that you have databases down, you need to be able to access the data on the front-end through APIs (Application Programming Interface). It is a way for the front-end to consume the data from the back-end.
Here's a fun tutorial on how to build your own API: Make Your Own API (Ania Kubow).
Authentication
If your site needs to keep track of users, you'll need to implement authentication. The most common ways to do this is by using OAuth or JavaScript Web Tokens (JWT).
To make things easier though, you can use authentication providers such as Auth0 or Firebase.
Resources:
- Simple React User Login Authentication with Auth0 (codeSTACKr)
- React Authentication Crash Course With Firebase (Web Dev Simplified)
Blockchain / Cryptocurrency
Blockchain is a type of database. But it's not like a typical database. It stores data in blocks and then chains them together in chronological order.
You can store any type of data in a block, but it's typically used as a ledger for transactions.
Bitcoin uses blockchain in a decentralized way so that no single person has control.
Decentralized blockchains are immutable. That means that the data cannot be changed. Once a transaction is recorded, it's permanent and everyone can see it.
This makes blockchain very secure.
You will see blockchain and cryptocurrencies continue to become more popular in 2021.
To learn more about these technologies visit:
Content Management Systems (CMS)
Content management systems allow you to easily manage the content of your site. If you are a freelancer, you will most definately want a good CMS for your customer to use so that they can easily update the content on their website themselves.
Traditional CMS's are Wordpress and Drupal.
In 2020, headless CMS's became very popular and will continue to be in 2021.
Headless CMS allow you to use whatever you want on the front-end. You are not tied to their platform. This gives you much more freedom to build your application.
Examples of headless CMS's:
Resources:
- How to make a WordPress website from scratch (Adrian Twarog)
- Getting Started with Sanity.io (James Q Quick)
- Build a Portfolio Site with Sanity.io and Gatsby (Jason Lengstorf)
- Strapi.js Crash Course (Traversy Media)
Deployment / DevOps
Deployment and DevOps involves understanding how to create and manage environments along with deploying applications to the web.
You'll need to understand how SSH works for connecting to remote environments.
You'll also need to learn about load balancing, monitoring, and security. Ensure that your sites are secure by installing SSL certificates.
GitHub Actions can also help with CI/CD (Continuous Integration / Continous Deployment)
Resources:
- DevOps Directive
- What are GitHub Actions? (Eddie Jaoude)
- Automatic Deployment with GitHub Actions (Traversy Media - Anson The Developer)
Hosting
You'll need to setup a hosting service. Depending on your needs, you can go with a static host like Netlify or GitHub Pages. These allow for easy deployment that can be integrated with GitHub. This way, every time you update your GitHub repo, your site will automatically rebuild and deploy.
If your application requires a true back-end environment you'll want a more traditional hosting service such as one of these:
Web Servers
Then, learn about web servers. Here are the popular ones:
- NGINX
- Apache
- MS IIS
Testing
Testing is necessary before deploying an application. The type and amount of testing needed depends on the complexity of your application.
These are some types of testing you'll want to learn:
- Unit
- Integration
- Functional
Serverless Architecture (FaaS)
FaaS stands for "Function as a Service". Serverless does not actually mean that there is no server. It just means that you do not manage the server. You only need a server to perform a minor function.
This means that there is less infrastructure for you to manage. The top serverless providers in my opinion are AWS Lambda and Netlify.
Resources:
Virtualization
Virtualization allows you to easily create and deploy containers, or "images", of enironments that are setup and ready to go. The leader in this is Docker.
Kubernetes allows you to automate container deployment, scaling, and management.
Resources:
Mobile Development
The traditional way to build a mobile application is by using languages such as Swift for iOS development and Java or Katlin for Android development. But if you've learned HTML, CSS, and JavaScript you can use these to build native mobile applications.
There are several technologies that are popular in mobile app development.
- React Native: Using your React skills you can easily build native mobile apps using React Native.
- Flutter: This uses the programming language Dart to build native mobile apps. You can even compile your existing Flutter code written in Dart into a web site!
- NativeScript: JavaScript, TypeScript, Angular, or Vue can be use with this to create native mobile apps.
- Ionic: You can build Native and Hybrid mobile apps with Ionic using React, Vue, or Angular.
Resources:
- React Native (William Candillon)
- Flutter (Flutter Explained)
- Flutter (Tadas Petra)
- Flutter (Robert Brunhage)
Desktop Development
You can also use your web development skills to build cross-platform desktop apps with Electron. Electron uses JavaScript, HTML, and CSS.
It uses Cromium and Node.js on the backend, and is compatible with Mac, Windows, and Linux.
Some popular apps that are built with Electron are Visual Studio Code, Twitch, Slack, Figma, and Discord.
Machine Learning / AI
AI and machine learning is widely used to imitate human intelligence and perform simple as well as complex functions like the ability to learn and analyze information, collect data, understand emotions of humans, or to solve complex business challenges and problems.
AI is everywhere, get used to it, itโs not going away.
Python is the main language that I think about when it comes to AI and ML. But there are great JavaScript libraries as well, like Tensorflow.js and Brain.js.
Resources:
Soft Skills & Personal Development
Something that often gets overlooked is soft skills, or a better name for it is people skills, along with personal development.
These are the top skills that you should invest effort in developing:
- Communication
- Teamwork
- Attention to Detail
- Problem Solving
- Work Ethic
- Time Management
- Adaptability
- Interpersonal Skills
- Creativity
- Leadership
Another huge issue that you will run into is loosing motivation and impostor syndrome. You need to surround yourself with people who are supportive to you and understand that you are not alone.
An amazing resource for motivation and dealing with impostor syndrome is Danny Thompson!! Check him out on YouTube and Twitter.
Honorable Mentions
There are a few technologies and that are not included in the roadmap but should still be mentioned.
Low-code / No-code
There are several platforms that provide the ability to create websites and applications with very little to no web development knowledge.
These are the platforms that scare a lot of developers because they believe their jobs will be taken by these technologies.
My advice is to not worry about these. The platforms themselves rely on web developers to create and maintain them.
These platforms are targeting an audience that needs very basic sites or applications. These cannot require advanced customization or have complex programming requirements.
Also, these applications do not scale very well. Once a company grows out of this basic app they will be looking for actual developers to help them.
VR / AR
Virtual reality and augmented reality are getting very popular. It is still very much in its infancy but will continue to grow in popularity in 2021.
Conclusion
We covered so many technologies in this article. If you made it to the end, your brain must be fried.
Remember, you don't have to learn everything we covered. Focus on the technology used in your current job or aspiring job. So, if your current employer uses Angular, focus on Angular. If the companies you are interviewing with use React, focus on React before your interviews.
Then you can learn other technologies in your spare time. (If you have any ๐) If you've gotten comfortable with JavaScript and you want to learn some Python in your spare time, then do that.
It's hard to stay up-to-date with current trends and technologies. But know that most companies are not using the most up-to-date technologies.
Learning something new takes time. It takes time to get good at something. So be patient, take your time, never stop learning, and never give up!
Check out the full video on my YouTube channel.
Help me out by liking this video and subscribing if you havenโt already.